Variables
A variable is a name you can use to hold a value. In Lua, a variable can have any data type, such as a number or a string.
Assignment
Assigning a value to a variable can be done using the = operator, like so:
x = 10 word = "Hello"
Variable x now has a value of the number 10, and word has a value of the string "Hello". If we print out these variables, we should see the value they have:
print(x) --> 10 print(word) --> Hello
A variable can be changed simply by assigning another value to it:
n = 12 print(n) --> 12 n = 24 print(n) --> 24
Even though n was assigned to a value of 12, it was overwritten by the second assignment of 24.
You can also use a variable as a value when assigning.
n = 78 x = n print(x) --> 78
When x was assigned to n, it's value became the value of n.
Remember! A variable is always on the left side of the = while a value is on the right side.
Names
The name you give a variable can only contain letters (uppercase and lowercase), numbers, or an underscore ( _ ). A variable name cannot be a reserved keyword or start with a number. Other than that, there are no restrictions.
LETTERS = "ABC" -- valid x86 = 1978 -- valid var_name = "variable" -- valid _ = "blank" -- valid if = 12 -- NOT valid! 16th = "Lincoln" -- NOT valid!
Multiple Assignment
Lua allows you to assign more than one value to more than one variable.
a, b, c = 1, 2, 3 print(a, b, c) --> 1 2 3
Each variable and each value is separated by a comma. Values to the right of the = are assigned to their corresponding variables to the left. The first variable is assigned to the first value, the second variable is assigned to the second value, and so on. The above example is the same as this:
a = 1 b = 2 c = 3 print(a, b, c) --> 1 2 3
Swapping
Multiple assignment is useful because it allows you to easily swap the values of two variables.
x, y = 8, 36 print(x, y) --> 8 36 x, y = y, x print(x, y) --> 36 8
In this example, x is assigned to the original value of y, and y is assigned to the original value of x.
Variable Scopes
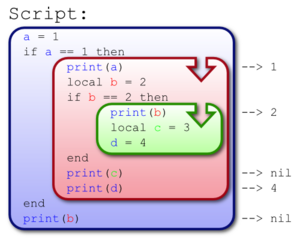
Sometimes you may want to limit the access of one variable to another. You can do this by allowing a variable to be visible only to others in the same area. These areas are called scopes. In normal Lua, there are two scopes, known as global and local.
Each block of code has its own scope. Since blocks can be nested, each scope may have an outer scope and an inner scope.
- The inner scope is a "child" of the current scope. The current scope cannot access variables in the inner scope.
- The outer scope is a the "parent" of the current scope. The current scope has access to any variables in the outer scope.
Global Variables
A global variable is a variable that is visible to all scopes of a script.
a = 1 if true then
print(a) --> 1 if true then a = 2 print(a) --> 2 end print(a) --> 2
end print(a) --> 2
Local Variables
A local variable is a variable that can only be accessed by other things in the same scope. A variable is made local by adding the keyword local before the variable:
local word = "Hello"
A local variable is not visible to things in its outer scope.
if true then -- a new block is created local b = 2 end print(b) --> nil
However, they are visible to things in its inner scope.
local b = 2 if true then print(b) --> 2 end
If a variable has the same name as one in its outer scope, the outer scope's value is overwritten in the inner scope, but not the outer scope.
a = 1 if true then local a = 2 print(a) --> 2 end print(a) --> 1