User:SoulStealer9875/Programming With Lua: Difference between revisions
>SoulStealer9875 No edit summary |
>SoulStealer9875 No edit summary |
||
Line 214: | Line 214: | ||
<nowiki>print("I need 1 chocolate bar in 5 days!")</nowiki> | <nowiki>print("I need 1 chocolate bar in 5 days!")</nowiki> | ||
<nowiki>print("I need", 1, "chocolate bar in", 5, "days!")</nowiki> | <nowiki>print("I need", 1, "chocolate bar in", 5, "days!")</nowiki> | ||
All three of the above examples will output: 'I need 1 chocolate bar in 5 days!'. | |||
}} | }} |
Revision as of 19:54, 4 September 2011
Introduction
What's your story?
- Are you the kind of person who wants to produce your own code?
- Do you want to understand Lua code?
- Are you someone who wants to know how code talks to the computer?
Well, if you want to write Lua code - I suggest you read this whole guide. This guide avoids the "of-course-you-already-know" assumption and describes Lua programming from scratch.
Icons used in this guide
- Tip. I use this when I want you to have some extra information; something helpful that will make you a bit more aware with coding in Lua.
- Warning. I use this when fiddly things come about. Things you may forget. For example, if I think you may capitalize a letter that may cause your script to break. I will use this icon.
Part 1
Chapter 1: Getting Ready
There's a few things you need to do before you start learning how to code in Lua. The first thing is to get ROBLOX Studio. If you don't already have this software, click here.
When you've downloaded ROBLOX Studio, or if you already have the official ROBLOX Software, click 'Start' -> All Programs -> Roblox -> ROBLOX Studio. This will open the software taking you into ROBLOX Studio.
When ROBLOX Studio finishes loading you will find a toolbar at the top. Find 'File' -> New. Selecting new will open up a new place, you will be required to use this while you read this guide.
Next, we need to insert a blank script. To do this, find 'Insert' in the toolbar -> Object. Selecting object will open up a dialog window with several objects you can insert into your game. Scroll around the dialog window until you find 'Script' then select OK.
If you don't already have the Explorer panel open, go to 'View' in the toolbar -> Explorer.
In the explorer panel, find '+ Workspace'. Press the + next to workspace then double click on 'Script'.
After double clicking on Script, you will be taken into a blank white window displaying the text by default: "print 'Hello World'". Highlight that text and delete it.
Exit out of the script's window and go to 'View' in the toolbar -> Output. This opens up the output window. This will come handy in later chapters when we learn to display text on it.
Chapter 2: Printing
Everyone knows social life is important, so why not be social to the computer? With Lua, and any other programming language, we can talk to the computer. Not literary, 'talk' to the computer. More like, allow the Lua programming language to turn the code into binary and send it to the system which reads the binary and does what's it told to do. Here's a diagram of how it works:
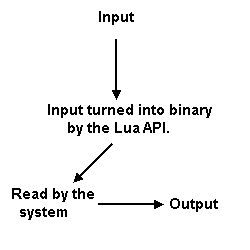
The diagram towards the left shows the input, this is the code that you type in. It is then turned into binary and sent off to the system. The system reads the binary code and executes it, otherwise known as the output.
Open up the script you inserted earlier. Before we deleted the default text which was "print 'Hello World'", if we were to run the script with the text on it and you had the output window open, the text "Hello World" would be displayed in it.
How is this? This is because the function "print" is executed and the text inside the quotation marks are displayed in the output window. This is called "printing text". There are other ways of printing text such as followed:
print('Hello World')
print("Hello World")
print [[Hello World]]
print([[Hello World]])
print "Hello World"
and so on.
Some people write a semicolon after these, but it doesn't make any difference; so don't worry about that.
- Do not type Print instead of print, this will break your code! It's print, with the lowercase 'p'.
You can also print arithmetic's such as followed:
print(2+2)
Would output: 4
print(2-2)
Would output: 0
print(2*2)
Would output: 4
You can use this to do your math homework!
print(16+(((2*9)/1)*6)%2)
Would output: 16
Chapter 3: Variables
First of all, what is a variable? A variable is something that holds data. You can have Numbers, Strings, Booleans and Objects.
You know what a number is, I hope. But what is a string? A string is something that holds words, letters... Generally any keyboard characters and can be found between speech marks, quotation marks and [[ and ]]'s. A boolean is something that contains two values, false and true. Object variables? What's an object variable? It's a variable that contains objects.
How does a variable 'think'? Well, here's a diagram of it:
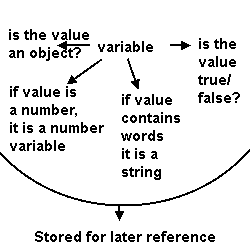
The diagram to the left shows the variable and what its job is. As an example, if the value of the variable was a number, it's a number value. If the value is either true or false, it's a boolean.
Well, how do you MAKE a variable? Let me assure you, making a variable is extremely easy. It's not like some other programming languages in which you have to define what type the variable is. Like in Java, you have to type:
Type Name = Value Boolean On = true
In Lua, we remove the type, so it gives us:
Name = Value
The 'Name' can be anything you want! Here's a couple of examples;
On = Value Hatred = Value Pizza = Value Friends = Value
What about the value? As we learned earlier, the value can be anything and the API can tell what type the variable is, so that saves us the extra work!
Here's a few examples;
On = false Hatred = true Pizza = true Friends = 1336
Cool! What else can we do with these 'variables'? Well, later on we're going to learn about the if statements. For now, we can use what we've learned to do our algebra homework! Oh, yes!
Wait a minute, I just remembered! I have some algebra homework. Oh no... Wait, with Lua, it's going to be FUN and EASY!! Here's what my homework is:
If the value of x is 5, what is the value of y if y is x + 3?
Well, I'm dumb. I don't know how to figure this out. It's too advanced for me. Fortunately, Lua is not dumb! So let's map this out.
'If the value of x is 5'. Hmm.. I know! I'll use a variables,
x = 5
'what is the value of y is y is x + 3?'. Does this mean I have to create a new variable for y? Well, in some cases yes, in other cases no. In this case we don't need to, but we will for future reference.
x = 5 y = 0
Now, we're going to do the arithmetic's.
x = 5 y = 0 y = x + 3
Now the value of y is no longer 0, it's x + 3, which is the same as saying 5 + 3, which equals 8. But we're not done yet! We have to get the computer to reply with the answer. So we're going to use the print function that prints the value of y, which is x + 3, which is 8.
x = 5 y = 0 y = x + 3 print(y)
Would output: 8. Yey! My algebra homework is complete. Let's think of easier ways of doing this:
x = 5 y = x + 3 print(y)
Well, it's one line smaller. Let's try and make it even smaller!
x = 5 print(x + 3)
... I'm still not happy. Can we slim this to one less line? I'd be happy to substitute the variable x!
print(5 + 3)
Hurrah! Now, we've covered the number variables. What about the others?
The second variable type we're going to learn about are Strings. Here's an example of one:
myString = "Hello World" print(myString)
The above example will print Hello World in the output window when the script is ran. Here's another example,
myString = "I just came from the world of zeros and ones!" print(myString)
Would print I just came from the world of zeros and ones!.
What about joining two variable strings together? We separate them by a comma:
myString = "Hello" mySecondString = "World" print(myString, mySecondString)
The above would result in 'Hello World'.
How about joining one string to another variable? Well, to do this we use two dots ( .. ) as found here:
myString = "World" print("Hello " .. myString)
Hmm.. What about two strings in between a variable?
myString = "Five" print("I have " .. myString .. " friends")
Would result in 'I have Five friends'.
More examples:
myNumber = 5 myString = "friends" myOtherString = "I have" print(myOtherString, myNumber, myOtherString)
Would result in: I have 5 friends
print("I need " .. 1 .. " chocolate bar in " .. 5 .. " days!") print("I need 1 chocolate bar in 5 days!") print("I need", 1, "chocolate bar in", 5, "days!")
All three of the above examples will output: 'I need 1 chocolate bar in 5 days!'.